Microcontroller Programming
Program an Arduino board to do something. Include code snippets in your documentation. Bring your circuit to class Tuesday and be ready to do a brief live demo.
I wanted to experiment with using LEDs for this week's assignment. I also think the potentiometer is a cool and neat component, so I wanted to use it, too. I used the motor driving class page as reference for how to use the potentiometer, and Sparkfun's LED tutorial as reference for the LEDs.
I made a progress-bar type of LED sequence. As the potentiometer is turned, more lights come on. As you turn it back, the lights turn back off.
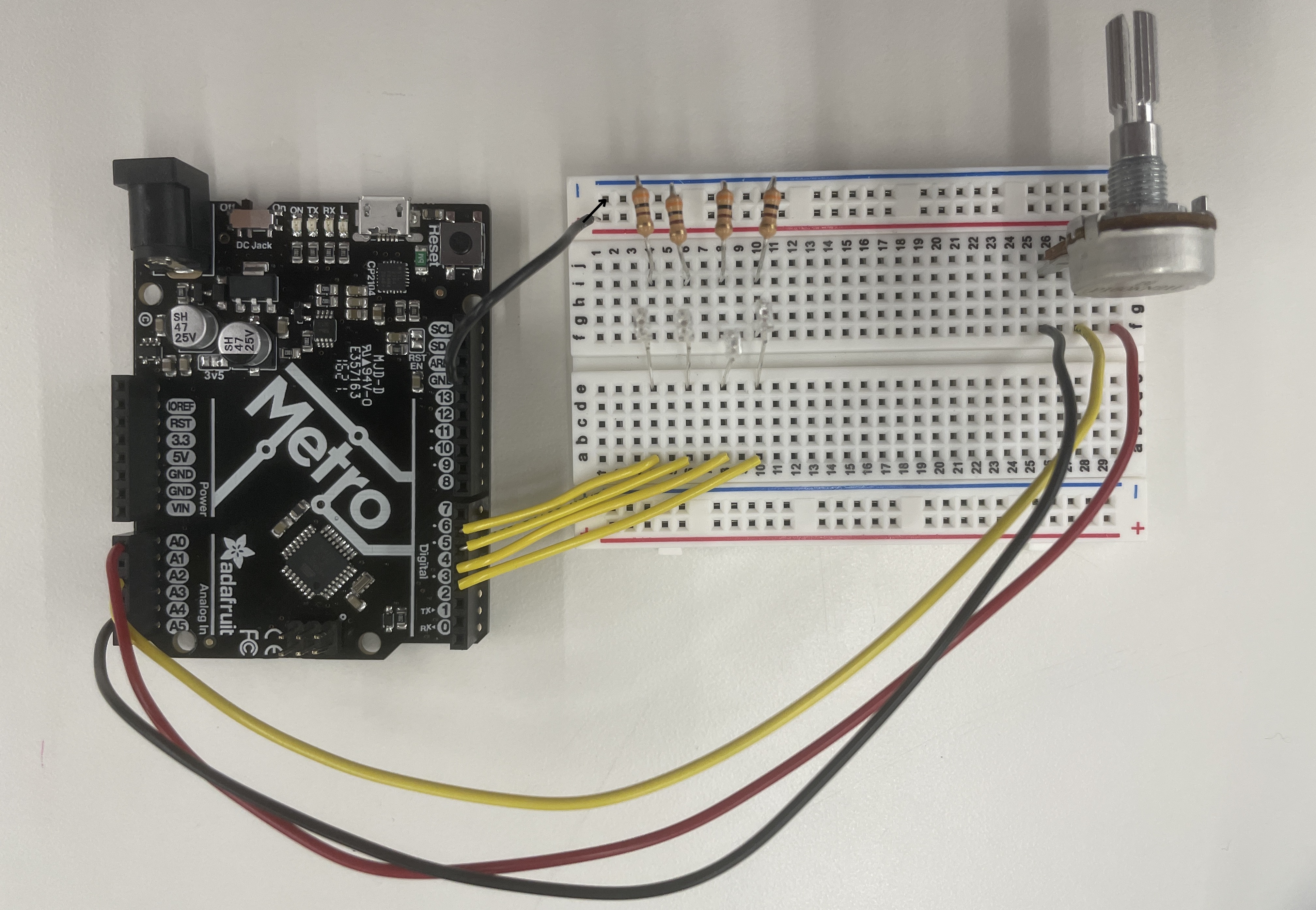
Final wiring. pretend the black ground wire didn't fall out of the breadboard :/
Each LED needs a resistor, a connection to ground, and independent connection to power from the Arduino pins. The LEDs are connected in series with the resistors all attaching to ground, and the positive side of the LED attaching to digital pins 3 through 6. The potentiometer is connected to A0, A2, and A4, just like the in-class example.
But in order to light up the LEDs based on the potentiometer, I needed to program the microcontroller. Code here is adapted from the in-class examples.
int leds[] = {3, 4, 5, 6};
//int leds[] = {6, 5, 4, 3}; // use this to make the lights go backwards
int numleds = 4; // because .length isn't here :(
void setup() {
// set LED pins to output and off
for (int i = 0; i < numleds; i++) {
pinMode(leds[i], OUTPUT);
digitalWrite(leds[i], LOW);
}
pinMode(A0, OUTPUT); //This will be GND for the potentiometer
pinMode(A4, OUTPUT); //This will be 3.3V for the pot
pinMode(A2, INPUT);
digitalWrite(A0, LOW);
digitalWrite(A4, HIGH);
}
void loop() {
// check the potentiometer value
int pot_value = analogRead(A2);
// map it to useful values representing the # of leds on
// since map() truncates, use numleds+1 instead of numleds
int led_value = map(pot_value, 0, 1023, 0, numleds+1);
// for each led in leds[]
for (int l = 0; l < numleds; l++) {
// if it should be on, turn it on
if (l < led_value) {
digitalWrite(leds[l], HIGH);
// otherwise turn it off
} else {
digitalWrite(leds[l], LOW);
}
}
// this feels like an appropriately speedy response time
delay(100);
}
Getting to this working code took a fair bit of debugging. Notably, the last light wouldn't turn on until I realized that map() truncates. Also, digital pin 1 simply doesn't work the way I intended, so I switched to using pins 3-6 to avoid the problem.
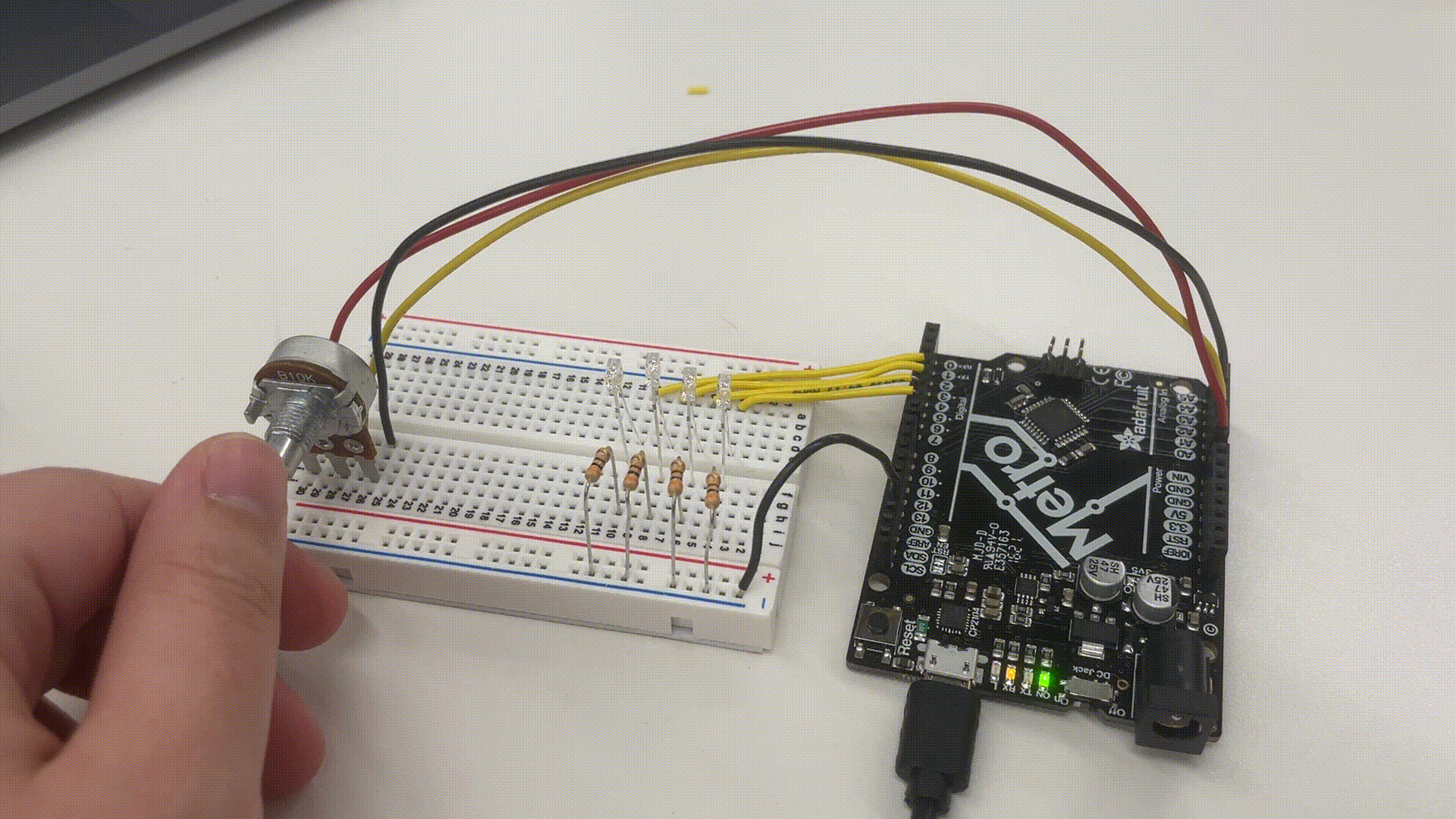
it works! this is with the "backwards" code configuration.